截图
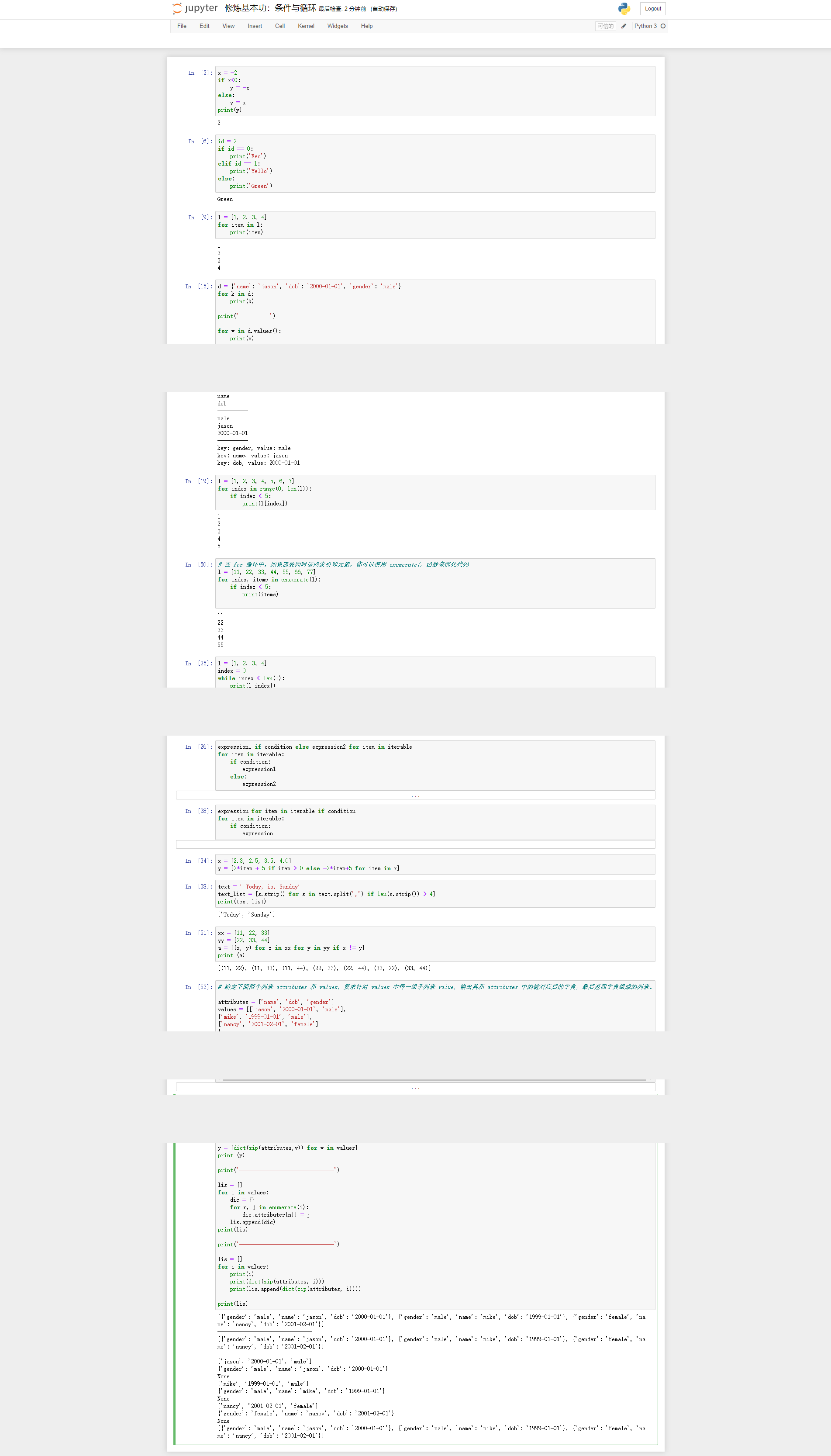
代码
#!/usr/bin/env python
# coding: utf-8
# In[3]:
x = -2
if x<0:
y = -x
else:
y = x
print(y)
# In[6]:
id = 2
if id == 0:
print('Red')
elif id == 1:
print('Yello')
else:
print('Green')
# In[9]:
l = [1, 2, 3, 4]
for item in l:
print(item)
# In[15]:
d = {'name': 'jason', 'dob': '2000-01-01', 'gender': 'male'}
for k in d:
print(k)
print('----------')
for v in d.values():
print(v)
print('----------')
for k, v in d.items():
print('key: {}, value: {}'.format(k, v))
# In[19]:
l = [1, 2, 3, 4, 5, 6, 7]
for index in range(0, len(l)):
if index < 5:
print(l[index])
# In[50]:
# 在 for 循环中,如果需要同时访问索引和元素,你可以使用 enumerate() 函数来简化代码
l = [11, 22, 33, 44, 55, 66, 77]
for index, items in enumerate(l):
if index < 5:
print(items)
# In[25]:
l = [1, 2, 3, 4]
index = 0
while index < len(l):
print(l[index])
index += 1
# In[26]:
expression1 if condition else expression2 for item in iterable
for item in iterable:
if condition:
expression1
else:
expression2
# In[28]:
expression for item in iterable if condition
for item in iterable:
if condition:
expression
# In[34]:
x = [2.3, 2.5, 3.5, 4.0]
y = [2*item + 5 if item > 0 else -2*item+5 for item in x]
# In[38]:
text = ' Today, is, Sunday'
text_list = [s.strip() for s in text.split(',') if len(s.strip()) > 4]
print(text_list)
# In[51]:
xx = [11, 22, 33]
yy = [22, 33, 44]
a = [(x, y) for x in xx for y in yy if x != y]
print (a)
# In[52]:
# 给定下面两个列表 attributes 和 values,要求针对 values 中每一组子列表 value,输出其和 attributes 中的键对应后的字典,最后返回字典组成的列表。
attributes = ['name', 'dob', 'gender']
values = [['jason', '2000-01-01', 'male'],
['mike', '1999-01-01', 'male'],
['nancy', '2001-02-01', 'female']
]
# expected output:
[{'name': 'jason', 'dob': '2000-01-01', 'gender': 'male'},
{'name': 'mike', 'dob': '1999-01-01', 'gender': 'male'},
{'name': 'nancy', 'dob': '2001-02-01', 'gender': 'female'}]
# In[57]:
attributes = ['name', 'dob', 'gender']
values = [['jason', '2000-01-01', 'male'],
['mike', '1999-01-01', 'male'],
['nancy', '2001-02-01', 'female']
]
y = [dict(zip(attributes,v)) for v in values]
print (y)
print('-------------------------------')
lis = []
for i in values:
dic = {}
for n, j in enumerate(i):
dic[attributes[n]] = j
lis.append(dic)
print(lis)
print('-------------------------------')
lis = []
for i in values:
print(i)
print(dict(zip(attributes, i)))
print(lis.append(dict(zip(attributes, i))))
print(lis)