代码
#!/usr/bin/env python
# coding: utf-8
# In[3]:
# 什么是字符串呢?字符串是由独立字符组成的一个序列
# 通常包含在单引号('')双引号("")或者三引号之中(''' '''或""" """,两者一样)
# 比如下面几种写法
name = 'jason'
city ='beijing'
text = "Welcome to 噜啦"
print(name)
print(city)
print(text)
# In[5]:
# Python 中单引号、双引号和三引号的字符串是一模一样的,没有区别
# 比如下面这个例子中的 s1、s2、s3 完全一样
s1 = 'hello'
s2 = "hello"
s3 = """hello"""
print(s1 == s2 == s3)
# In[9]:
s = 'a\nb\tc'
print(s)
# In[19]:
name = 'jason'
print(name[0])
print(name[1:3])
print ("\n")
for char in name:
print (char)
# In[21]:
# Python 的字符串是不可变的(immutable)。
# 因此,用下面的操作,来改变一个字符串内部的字符是错误的,不允许的
a = 'hello'
a[0] = 'H'
# In[24]:
# Python 中字符串的改变,通常只能通过创建新的字符串来完成。
a = 'hello'
a = 'H' + a[1:]
print(a)
# In[26]:
# 使用加法操作符'+='的字符串拼接方法
str1 = 'aaaa'
str2 = 'bbbb'
str1 += str2
print(str1)
# In[34]:
s = ""
for n in range(0, 1000):
s += (str(n) + ",")
print (s)
# In[39]:
# 分割函数 split 把字符串按照 separator 分割成子字符串
# 并返回一个分割后子字符串组合的列表
def query_data(namespace, table):
"""
given namespace and table, query database to get corresponding
data
"""
path = 'hive://ads/training_table'
namespace = path.split('//')[1].split('/')[0]
print(namespace)
table = path.split('//')[1].split('/')[1]
print(table)
# In[60]:
# string.strip(str),表示去掉首尾的 str 字符串;
# string.lstrip(str),表示只去掉开头的 str 字符串;
# string.rstrip(str),表示只去掉尾部的 str 字符串。
# 从文件读进来的字符串中,开头和结尾都含有空字符,我们需要去掉它们,就可以用 strip() 函数
s = ' my name is jason '
s.strip()
# In[63]:
id = '007'
name = '噜啦'
print('No data available for person with id: {}, name: {}'.format(id, name))
# In[ ]:
# 下面的两个字符串拼接操作,你觉得哪个更优呢?
# In[5]:
import time
start_time = time.perf_counter()
s = ''
for n in range(0, 5000000):
s += str(n)
end_time = time.perf_counter()
print('Time elapse: {}'.format(end_time - start_time))
# In[3]:
import time
start_time = time.perf_counter()
s = []
for n in range(0, 500000):
s.append(str(n))
''.join(s)
end_time = time.perf_counter()
print('Time elapse: {}'.format(end_time - start_time))
# In[4]:
import time
start_time = time.perf_counter()
s = ''.join(map(str, range(0, 500000)))
end_time = time.perf_counter()
print('Time elapse: {}'.format(end_time - start_time))
截图
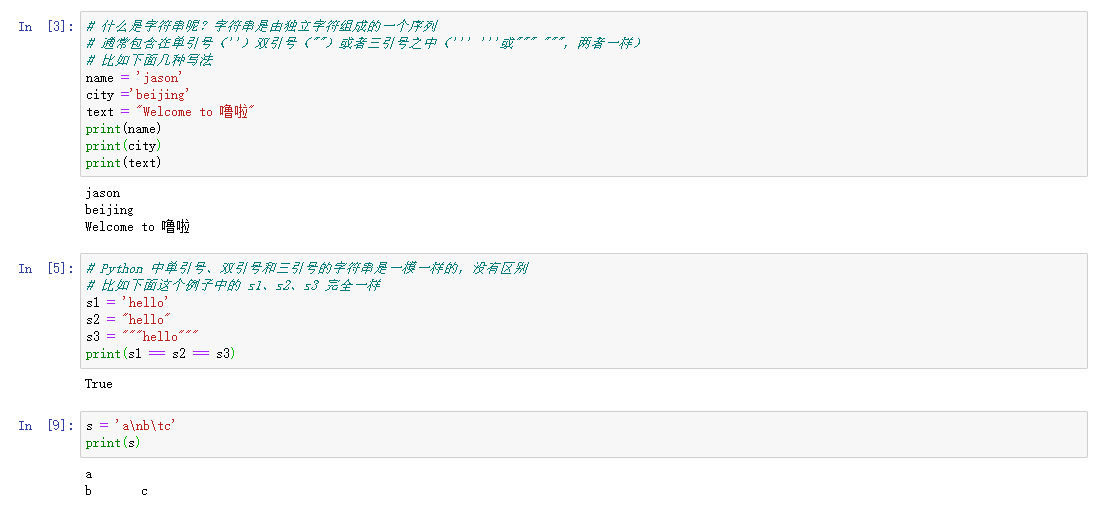